Understanding Data Structures: A Beginner’s Guide for Students
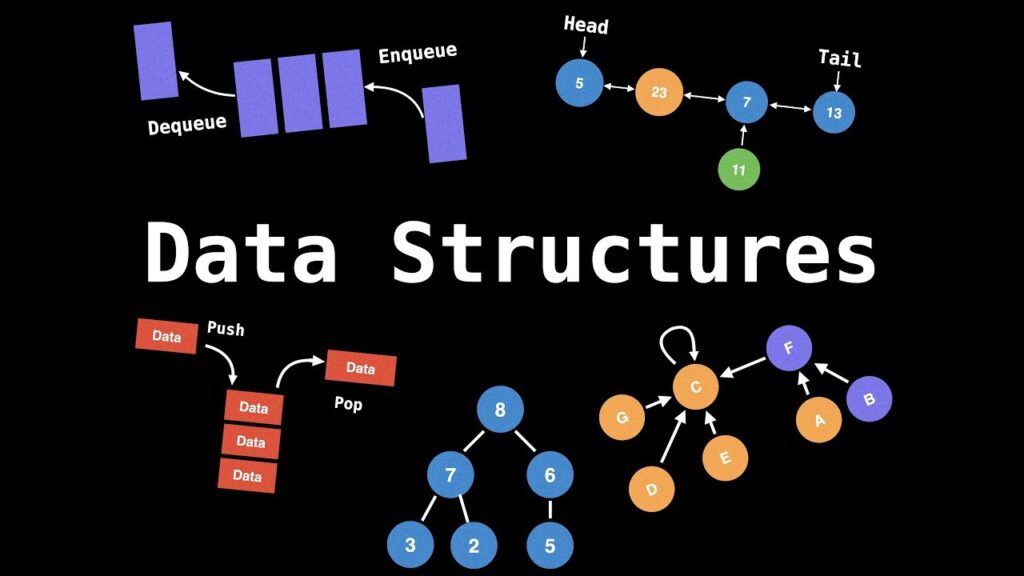
Data structures are a foundational concept in computer science that every student must grasp, especially when tackling computer science assignments. They are crucial for organizing, managing, and storing data efficiently, which is essential for writing effective and optimized code. This beginner’s guide aims to provide an overview of basic data structures, their importance, and practical tips specifically for students studying computer science. The examples in this guide are provided using Python, a popular language in computer science education.
What are Data Structures?
Data structures are ways to store and organize data in a computer so that it can be accessed and modified efficiently. They are the backbone of software applications and are used in various aspects of computing, from database management to algorithm implementation.
Why are Data Structures Important for Students?
Understanding data structures is crucial for several reasons:
- Efficiency: Proper use of data structures can significantly improve the efficiency of your code.
- Scalability: Efficient data management allows programs to handle larger datasets.
- Problem-Solving: Many algorithmic problems can be solved more easily and efficiently with the right data structures.
- Foundation for Advanced Topics: Mastery of data structures is essential for learning more advanced computer science topics and completing complex computer science assignments.
Common Data Structures
Here’s a look at some of the most common data structures that every computer science student should know:
1. Arrays
- Definition: A collection of elements, each identified by an index or key.
- Usage: Useful for storing fixed-size sequences of elements.
- Example: Storing a list of student names in Python.
2. Linked Lists
- Definition: A linear collection of elements where each element points to the next, forming a sequence.
- Usage: Efficient for insertions and deletions; useful in scenarios where the size of the data structure changes frequently.
- Example: Implementing a queue or a stack in Python.
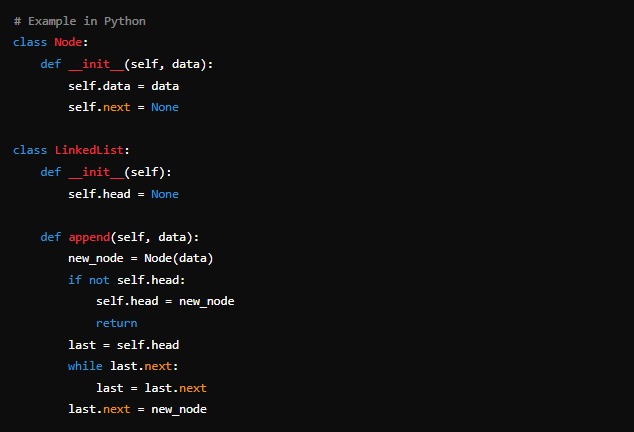
3. Stacks
- Definition: A linear data structure that follows the Last In, First Out (LIFO) principle.
- Usage: Useful for undo mechanisms, parsing expressions, and backtracking algorithms.
- Example: Browser history, undo feature in text editors, implemented in Python.

4. Queues
- Definition: A linear data structure that follows the First In, First Out (FIFO) principle.
- Usage: Useful for managing tasks in order, such as scheduling and handling requests.
- Example: Print queue, task scheduling, implemented in Python.
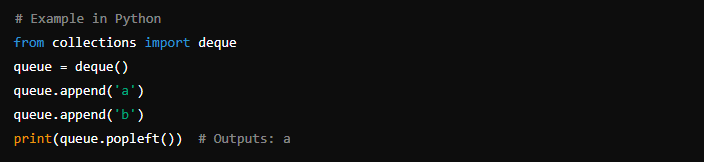
5. Trees
- Definition: A hierarchical data structure consisting of nodes, with a single node as the root and sub-nodes forming the branches.
- Usage: Useful for representing hierarchical data, such as file systems, organization charts, and databases.
- Example: Binary search trees, decision trees, implemented in Python.
6. Graphs
- Definition: A collection of nodes (vertices) and edges connecting them.
- Usage: Useful for representing networks, such as social networks, communication networks, and transport systems.
- Example: Friend connections in social media, shortest path algorithms, represented in Python.
Tips for Students
1. Practice Regularly
Consistent practice is key to mastering data structures. Use platforms like LeetCode, HackerRank, and CodeSignal to solve problems and improve your computer science skills.
2. Understand the Basics
Before diving into complex data structures, ensure you have a solid understanding of basic ones like arrays and linked lists. This foundation will make learning advanced structures easier.
3. Use Visual Aids
Visualizing data structures can help you understand how they work. Use tools like VisuAlgo and GraphViz to create visual representations of data structures and algorithms.
4. Implement from Scratch
Try implementing data structures from scratch in Python or your preferred programming language. This hands-on approach will deepen your understanding and improve your coding skills.
5. Study Real-World Applications
Look at how data structures are used in real-world applications. This can provide context and make the learning process more interesting and relevant.
Conclusion
Understanding data structures is fundamental to excelling in computer science. By familiarizing yourself with the basics and regularly practicing, you can develop a strong foundation that will serve you well in advanced studies and professional work. Keep exploring, practicing, and applying these concepts, and you’ll find yourself mastering data structures in no time.
For more resources and assistance with your computer science assignments, visit Research Hub Online.